In an era dominated by precision, real-time interaction, and global mobility, the ambition to develop a fully-detailed mapping application is no longer a dream—it’s a technical reality. This article explores the end-to-end development of a comprehensive global map app using .NET and Golang, detailing every structure: roads, rivers, oceans, lakes, terrain, and even nuanced geological features.
Our purpose is to break away from generic “map tutorials” and walk through the deeply integrated, data-centric, multi-layered structure necessary to simulate the Earth’s geography at a near-realistic scale.
Sample Code: Key Components of the Map App
1. Golang: Tile Server (Simplified)
package main
import (
"log"
"net/http"
)
func tileHandler(w http.ResponseWriter, r *http.Request) {
// Placeholder logic to serve vector tile
w.Header().Set("Content-Type", "application/x-protobuf")
// Load .pbf or generate dynamically here
w.Write([]byte("tile-binary-data"))
}
func main() {
http.HandleFunc("/tiles/", tileHandler)
log.Println("Starting tile server on :8080")
log.Fatal(http.ListenAndServe(":8080", nil))
}
2. .NET (C#): ASP.NET Core API Endpoint for Geocoding
[ApiController]
[Route("api/[controller]")]
public class GeocodeController : ControllerBase
{
[HttpGet("reverse")]
public IActionResult ReverseGeocode(double lat, double lon)
{
// Placeholder reverse geocoding logic
var place = new { Latitude = lat, Longitude = lon, Name = "Sample Place" };
return Ok(place);
}
}
3. .NET MAUI: Display Map Tile Layer
<ContentPage xmlns="http://schemas.microsoft.com/dotnet/2021/maui"
xmlns:x="http://schemas.microsoft.com/winfx/2009/xaml"
x:Class="MapApp.Pages.MapPage">
<WebView x:Name="MapWebView" Source="https://yourdomain.com/map-view.html" />
</ContentPage>
4. Golang: Generate Elevation Data from DEM
func interpolateElevation(x, y int, data [][]float64) float64 {
// Bilinear interpolation logic
return (data[x][y] + data[x+1][y] + data[x][y+1] + data[x+1][y+1]) / 4
}
5. Shared Architecture: Database Query (PostGIS)
SELECT name, ST_AsGeoJSON(geom)
FROM rivers
WHERE ST_Intersects(geom, ST_MakeEnvelope($1, $2, $3, $4, 4326));
This sample code illustrates the primary architecture: Go handles heavy geospatial processing and tiles, while .NET manages APIs and frontend rendering. Expand each with security, error handling, and scaling for production readiness.
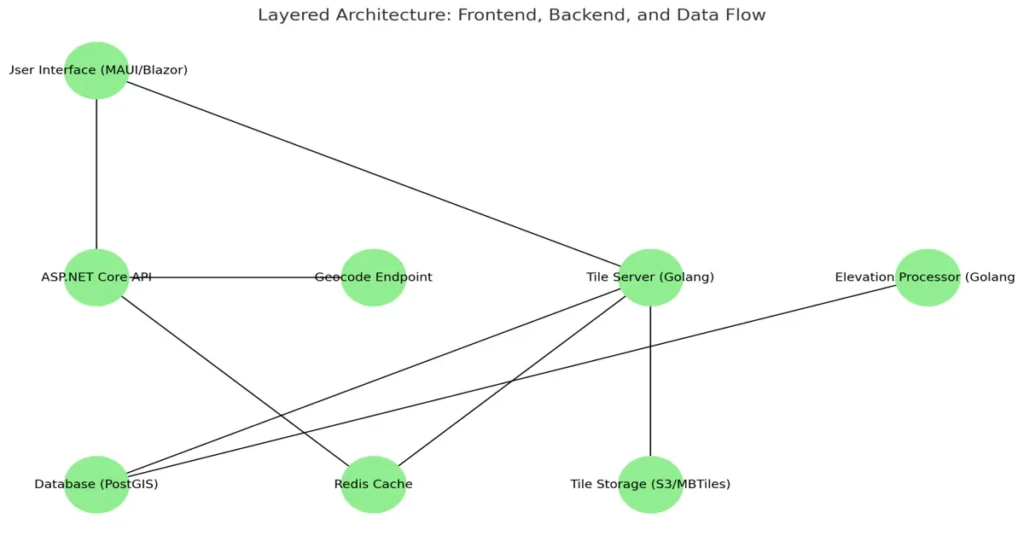
Vision of the App: A Planetary Model in Code
This is not about pins on a map. The app we envision:
- Renders street-level details
- Integrates water bodies, elevation, and tectonic features
- Allows offline and online modes
- Supports routing, geocoding, reverse-geocoding
- Operates on web and native platforms
- Offers high performance with scalable backend services
To achieve this, we need a deliberate architecture that leverages .NET for service layers and frontend integration, and Golang for high-throughput data processing and geo-computation.
1. Data: Foundation of the Map
Global Geospatial Datasets
To model the planet, we need multi-resolution datasets:
- OpenStreetMap (OSM): Roads, buildings, and administrative boundaries
- Natural Earth: Political boundaries, terrain, rivers, lakes
- Shuttle Radar Topography Mission (SRTM): Elevation and terrain
- HydroSHEDS: Rivers and watersheds
Use Go services to download, parse, and index this data into structured formats like GeoJSON or vector tiles.
2. System Architecture Overview
Frontend:
- .NET MAUI / Blazor for cross-platform mobile and desktop GUI
- Dynamic tile rendering using SkiaSharp or WebGL
Backend:
- Golang Services
- Tile server for vector/raster rendering
- Topology processor for rivers and elevation
- Spatial routing engine (e.g., Dijkstra or A*)
- .NET Web API
- User management, location tracking, and configuration
- Geocoding endpoints
Data Layer:
- PostgreSQL + PostGIS for spatial queries
- Redis for caching tiles and search
- S3-compatible storage for tile hosting
3. Go Modules for Geo Processing
Golang’s concurrency and performance make it ideal for geographic processing:
Tile Generation Module
- Converts GIS data to vector tiles (MVT format)
- Uses
go-geom
andgo-mbtiles
packages - Implements quad-tree or Hilbert curve indexing
Elevation Engine
- Parses DEM (Digital Elevation Models)
- Interpolates elevation data using bilinear methods
- Returns slope and altitude for any (lat, lon)
Hydrology Processor
- Traces river paths using flow direction data
- Connects river basins with nearby water bodies
- Encodes watersheds for simulation modeling
4. .NET Layers for Application Logic
.NET adds robustness, abstraction, and rich UI capabilities:
ASP.NET Core API
- Token-based authentication
- User-specific map layers (heatmaps, tracks, pins)
- Location history and analytics
Blazor / MAUI Frontend
- Real-time rendering of map tiles
- Zoom and pan logic
- Layer toggle (roads, rivers, elevation, cities)
- UI for routing, bookmarks, and terrain profiles
Dependency Injection and Modularity
- Use
IServiceCollection
for DI - Repositories for data access
- Services for user preferences and routes
5. Key Features and How to Build Them
A. Real-Time Routing
- Use A* or Dijkstra algorithm
- Heuristics based on road class, elevation gain
- Go service handles route calculation
- .NET client sends requests and renders path
B. Terrain Visualization
- Color ramp based on elevation
- Use SkiaSharp or Three.js to render 3D terrain views
- Combine hillshade and contour layers
C. Hydrological Mapping
- Draw rivers and tributaries with flow direction arrows
- Model upstream/downstream relationships
- .NET API allows querying watershed statistics
D. Offline Support
- Bundle tiles in SQLite or MBTiles format
- Allow Go service to serve tiles from local store
- Enable selective download regions
E. Advanced Search
- Full-text search with PostGIS + trigram index
- Fuzzy match for city, street, waterbody
- Custom geocoder in .NET with Redis caching
6. Optimization Techniques
Caching
- Redis for tile and query caching
- Client-side caching in Blazor with IndexedDB
Tile Compression
- Use gzip or vector tile compression (PBF)
- Split tiles by zoom level and popularity
Progressive Rendering
- Load base layers first (boundaries, water)
- Lazy-load roads, terrain, and labels
- Pre-fetch neighbor tiles on pan/zoom
7. Security and Access Control
Authentication
- OAuth 2.0 with JWT tokens
- Role-based access for editing and uploads
Rate Limiting
- Golang reverse proxy applies token bucket or leaky bucket algorithms
- Prevent tile flooding or abusive requests
Data Licensing Compliance
- Embed attribution logic into every tile render
- Provide open data disclaimers in UI
8. Performance and Scalability
Horizontal Scaling
- Stateless Golang tile/render services
- Use Kubernetes with horizontal pod autoscaling
CDN Integration
- Serve tiles via CloudFront or equivalent
- Edge cache heavy-traffic regions
Logging and Monitoring
- Prometheus for Go metrics
- Serilog for .NET logs
- Grafana dashboards for uptime and performance
9. AI and Predictive Layers (Optional)
Flood Risk Mapping
- Integrate rainfall data and simulate river overflow
- Model prediction using Go-based simulations
Traffic Prediction
- Time series from user telemetry
- ML model trained in .NET and inferenced in Go service
Elevation-Aware Routing
- Preference for flat routes in cycling/hiking
- Compute gradient penalty during pathfinding
10. Final Thoughts: Building the Future of Maps
Creating a planetary-scale map application is a grand challenge. It requires depth in:
- Geospatial computation
- System architecture
- Visualization
- Performance engineering
But with the dual strengths of .NET’s productivity and UI richness and Go’s raw speed and concurrency, it is entirely achievable – Best Map App.
The future of mapping is not static. It’s interactive, intelligent, and tailored to user context—from a city biker to an oceanographer.
This article is not a blueprint to follow line by line, but a strategy. A vision. An invitation to explore how modern technology can literally map the world—and how developers like you can build it – Best Map App.
Read:
The 30 Most Asked Interview Questions in .NET and Golang: A Developer’s Guide
The History of .NET and Golang: Parallel Journeys in Software Evolution
Deep Inside of the .NET and Golang Platforms: A Technical Exploration
A Comprehensive List of Where .NET and Golang Are Used: Mapping Real-World Applications
The Efficiency of .NET and Go (Golang) Coding: A Contemporary Technical Analysis